Mastering Vue Applications with the Quasar Framework
Written on
Chapter 1: Introduction to Quasar
Quasar is a widely-used Vue UI library designed to help developers create visually appealing Vue applications. In this guide, we will explore how to effectively utilize the Quasar UI library to build robust Vue applications.
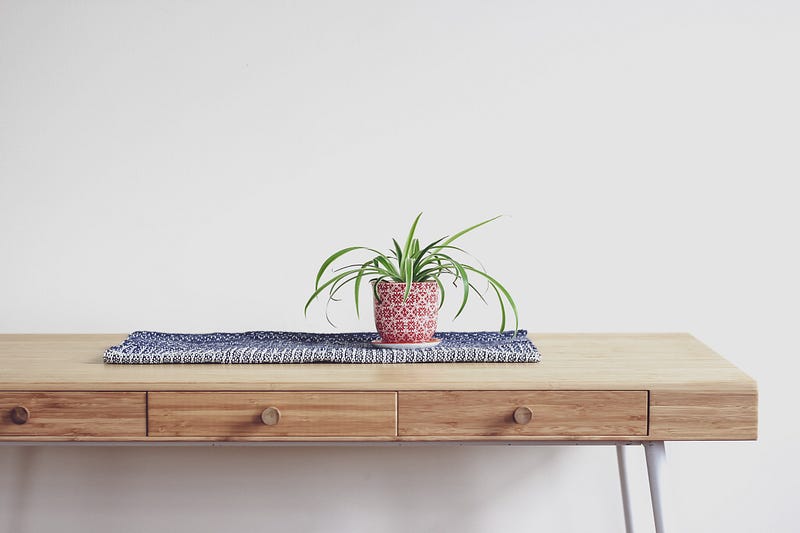
Section 1.1: Utilizing the q-table Component
Quasar's q-table component can automatically detect the column headers based on the data provided. For example, the following HTML snippet demonstrates how to implement this feature:
<!DOCTYPE html>
<html>
<head>
<link
rel="stylesheet"
type="text/css"
/>
<link
rel="stylesheet"
type="text/css"
/>
</head>
<body class="body--dark">
<div id="q-app">
<q-layout
view="lHh Lpr lFf"
container
style="height: 100vh;"
class="shadow-2 rounded-borders"
>
<div class="q-pa-md">
<q-table title="Treats" :data="data" row-key="name"> </q-table></div>
</q-layout>
</div>
<script>
const data = [
{ name: "Frozen Yogurt", calories: 159, fat: 6.0, calcium: "14%" },
{ name: "Ice cream sandwich", calories: 237, fat: 9.0, calcium: "8%" },
{ name: "Eclair", calories: 262, fat: 16.0, calcium: "6%" },
{ name: "Honeycomb", calories: 408, fat: 3.2, calcium: "0%" },
{ name: "Donut", calories: 452, fat: 25.0, calcium: "2%" },
{ name: "KitKat", calories: 518, fat: 26.0, calcium: "12%" }
];
new Vue({
el: "#q-app",
data: {
data}
});
</script>
</body>
</html>
In this setup, the property names are automatically capitalized to create the column headers.
Section 1.2: Creating a Sticky Header
To keep the table header fixed while scrolling, we can apply specific CSS styles. Here’s how to achieve a sticky header:
<!DOCTYPE html>
<html>
<head>
<link
rel="stylesheet"
type="text/css"
/>
<link
rel="stylesheet"
type="text/css"
/>
<style>
.sticky-header-table {
height: 300px;}
.sticky-header-table .q-table__top,
.sticky-header-table .q-table__bottom,
.sticky-header-table thead tr:first-child th {
background-color: yellow;}
.sticky-header-table thead tr th {
position: sticky;
z-index: 1;
}
.sticky-header-table thead tr:first-child th {
top: 0;}
.sticky-header-table.q-table--loading thead tr:last-child th {
top: 48px;}
</style>
</head>
<body class="body--dark">
<div id="q-app">
<q-layout
view="lHh Lpr lFf"
container
style="height: 100vh;"
class="shadow-2 rounded-borders"
>
<div class="q-pa-md">
<q-table
class="sticky-header-table"
title="Treats" :data="data"
row-key="name"
>
</q-table>
</div>
</q-layout>
</div>
<script>
const data = [
{ name: "Frozen Yogurt", calories: 159, fat: 6.0, calcium: "14%" },
{ name: "Ice cream sandwich", calories: 237, fat: 9.0, calcium: "8%" },
{ name: "Eclair", calories: 262, fat: 16.0, calcium: "6%" },
{ name: "Honeycomb", calories: 408, fat: 3.2, calcium: "0%" },
{ name: "Donut", calories: 452, fat: 25.0, calcium: "2%" },
{ name: "KitKat", calories: 518, fat: 26.0, calcium: "12%" }
];
new Vue({
el: "#q-app",
data: {
data}
});
</script>
</body>
</html>
To create a sticky header, we set the th element's position to sticky. Additionally, we defined the table's height to 300px to allow for scrollable content.
This video, "The Blue Standard 101 - Building Quasar Apps That Scale," provides insights into building scalable applications using Quasar.
In this tutorial, "Quasar Vue.js Tutorial - Let's Build An App!" you will learn how to build an application with Quasar and Vue.js.
Conclusion
In summary, we can enhance our tables with various styles, including sticky headers, and we can utilize Quasar's q-table component without needing to define columns explicitly.