Animating with the react-spring Library: Trail and Transition Components
Written on
Chapter 1: Introduction to react-spring
The react-spring library simplifies the process of adding animations to your React applications. With its intuitive components, you can create fluid animations with minimal effort.
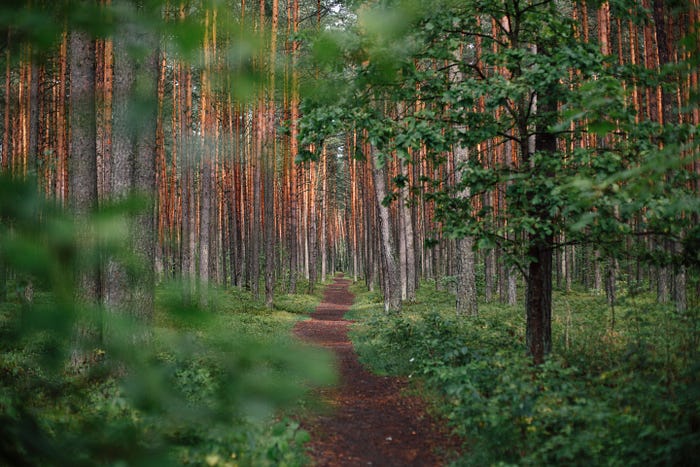
In this guide, we will explore how to begin utilizing the react-spring library, focusing on two key components: Trail and Transition.
Section 1.1: Understanding the Trail Component
The Trail component is designed to animate a list's first item, creating a visual effect where subsequent items follow in a graceful sequence.
For instance, you can implement it as follows:
import React from "react";
import { Trail } from "react-spring/renderprops";
const items = [
{ text: 1, key: 1 },
{ text: 2, key: 2 },
{ text: 3, key: 3 }
];
export default function App() {
return (
<Trail
items={items}
keys={item => item.key}
from={{ transform: "translate3d(0,-40px,0)" }}
to={{ transform: "translate3d(0,0px,0)" }}
>
{item => props => <div style={props}>{item.text}</div>}</Trail>
);
}
In this example, the Trail component receives an array of items to display. The keys function returns the key for each item. The from and to properties define the styles at the start and end of the animation, respectively. Consequently, you'll witness the numbers gracefully slide into view when the component mounts.
Section 1.2: Exploring the Transition Component
The Transition component allows for animating the lifecycle of components as they mount, unmount, or change.
Here’s how you can use it:
import React from "react";
import { Transition } from "react-spring/renderprops";
const items = [
{ text: 1, key: 1 },
{ text: 2, key: 2 },
{ text: 3, key: 3 }
];
export default function App() {
return (
<Transition
items={items}
keys={item => item.key}
from={{ transform: "translate3d(0,-40px,0)" }}
enter={{ transform: "translate3d(0,0px,0)" }}
leave={{ transform: "translate3d(0,-40px,0)" }}
>
{item => props => <div style={props}>{item.text}</div>}</Transition>
);
}
In this case, the Transition component also takes an array of items. The from property sets the initial animation styles, while enter and leave define the styles for when items appear and disappear. You’ll see the numbers smoothly slide down the screen upon rendering.
Additionally, the Transition component can be utilized to animate toggling between different components. Here's an example:
import React, { useState } from "react";
import { Transition } from "react-spring/renderprops";
export default function App() {
const [toggle, set] = useState(false);
return (
<div>
<button onClick={() => set(!toggle)}>Toggle</button>
<Transition
items={toggle}
from={{ opacity: 0 }}
enter={{ opacity: 1 }}
leave={{ opacity: 0 }}
>
{toggle => (props) =>
toggle ? (
<div style={props}>😄</div>) : (
<div style={props}>🤪</div>)
}
</Transition>
</div>
);
}
In this example, the useState hook manages the toggle state. The button updates this state, which in turn dictates which emoticon is displayed. The enter and leave properties control the fade-in and fade-out effects.
Conclusion
The Trail component is excellent for creating a cascading animation effect for a list's first item, while the Transition component allows you to manage the animation of component lifecycles seamlessly.
Chapter 2: Additional Resources
To further enhance your understanding of react-spring, check out these helpful video tutorials:
Learn how to implement animations using react-spring in this comprehensive video guide.
Follow along with this walkthrough to master the art of animating with react-spring.