Unlock the Power of JavaScript Destructuring for Cleaner Code
Written on
Chapter 1: Introduction to Destructuring
JavaScript developers can enhance their coding efficiency and clarity by harnessing the full potential of the language. One particularly underutilized feature that offers flexible value assignment from arrays and objects is destructuring. Let’s explore this powerful yet often overlooked tool to elevate your coding proficiency!
Section 1.1: Understanding Object Destructuring
Destructuring allows for the straightforward extraction of object properties into separate variables in a single operation. For instance, consider the following settings object:
const settings = {
notifications: true,
theme: 'dark'
};
We can assign the values to variables using destructuring:
const {notifications, theme} = settings;
console.log(notifications); // true
console.log(theme); // 'dark'
This method is efficient and eliminates the need for repetitive dot notation!
Subsection 1.1.1: Visualizing Object Destructuring
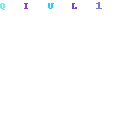
Section 1.2: Mastering Array Destructuring
In a similar manner, elements from arrays can be unpacked into individual variables:
const languages = ['JavaScript', 'Python', 'C++'];
const [first, second] = languages;
console.log(first); // JavaScript
console.log(second); // Python
This method is succinct and avoids the need for index references!
Chapter 2: Destructuring in Function Parameters
Destructuring truly shines when utilized in function parameter passing:
const person = {
name: "Kyle",
age: 24
}
function print({name, age}) {
console.log(name, age);
}
print(person); // Kyle 24
The function seamlessly receives cleanly destructured variables, removing any unnecessary clutter.
Section 2.1: Advantages of Using Destructuring
Destructuring offers several key benefits:
- Concise Value Assignment: Access properties and elements without redundancy.
- Parameter Passing Clarity: Clearly pass context variables.
- Scope Safety: Minimize the risk of variable name conflicts.
- Compatibility with Functions: Easily pass and return destructured parameters.
- Versatile Usage: Flexibly mix and match array and object destructuring.
Start using destructuring today to streamline your variable assignments!
Chapter 3: Practical Tips for Effective Destructuring
Here are a few parting tips to make the most of destructuring:
- Use destructuring for constants, function parameters, and return values.
- Apply array destructuring to assign elements meaningful names.
- Implement default and rest parameters for added flexibility.
- Embrace destructuring within function parameters for better clarity.
- Keep it simple at first — gradually introduce complexity.
Destructuring can transform the way you handle value assignments in JavaScript, turning a cumbersome process into a more enjoyable one. Unlock your coding efficiency today with this invaluable feature!