Understanding Type Parameters in Julia: A Comprehensive Guide
Written on
Chapter 1: Introduction to Type Parameters
Welcome back to the Comprehensive Julia Tutorial! In this session, we will delve into a crucial aspect of both dispatch and typing in Julia: Type Parameters. For those who are just starting with Julia, the concept of type parameters may seem overwhelming at first. The unusual syntax involving curly braces {} can be intimidating, but fear not! With a bit of guidance, you can grasp the fundamentals in no time, and that's exactly what I aim to share today.
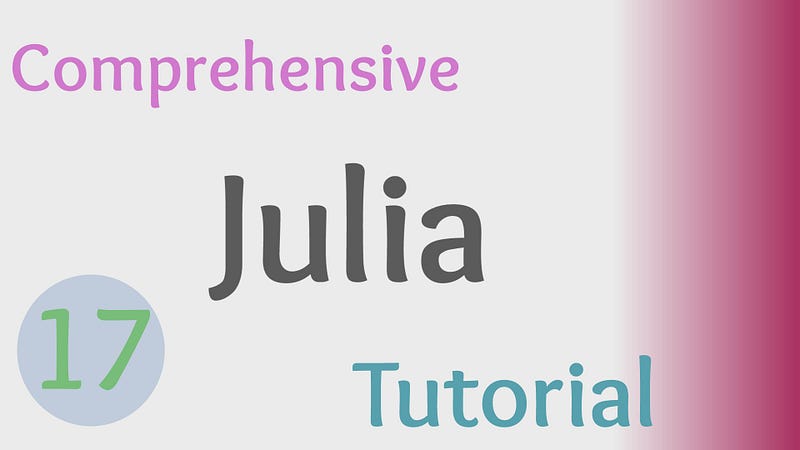
Chapter 1.1: The Role of Parameters
Type parameters exclusively utilize {} as their syntax, which previously also represented vector syntax. I misspoke in the video when I referred to dictionaries, as that term is more aligned with Python. In Julia, parameters are classified under the type Union, which can be expressed as a tuple and usually consists of a type or a symbol.
A quintessential example of type parameters in action is Julia’s vector typing. For instance, when we create a vector with homogeneous types:
x = [5, 10, 15]
This generates a vector that explicitly holds that type:
3-element Vector{Int64}:
510
15
Here, the type of each element is specified within the parameter. This not only boosts performance but also facilitates dispatching with various vector types. Consider the implications of this for data science: if you are working with both a categorical feature that needs encoding and a continuous feature that requires scaling, having the categorical feature as a String allows you to write a single function for both vectors, executing them in the same context.
Chapter 1.2: The Beauty of Multiple Dispatch
The real elegance of parameters shines through their relationship with multiple dispatch. When dealing with parameters, we can view them as an integral part of multiple dispatch. Consider the following function:
function printthis(x::Vector{Int64})
print(x)
end
Here, the argument x is specified as a Vector{Int64}, matching the global x, allowing it to be passed seamlessly as an argument:
printthis(x) # Outputs: [5, 10, 15]
Attempting to print a Vector{String} would result in a method error, as it is explicitly defined for Vector{Int64}:
y = ["String", "string2"]
printthis(y) # MethodError: No Method matching ...
We can also configure a parameter to accept a specific super-type, enabling us to work with any two types that share a common abstract relation. For instance:
function printthis(x::Vector{<:Number})
print(x)
end
Or even for any type:
function printthis(x::Vector{<:Any})
print(x)
end
Chapter 1.3: Exploring Function Parameters
Parameters play a fundamental role in all functions in Julia, determining the types of arguments for each method. The multiple dispatch mechanism in Julia refers back to a field known as sig in each method, allowing us to introspect the parameters for deeper insight:
methods(printthis)[1].sig.parameters[2] # Outputs: Vector{Int64} (alias for Array{Int64, 1})
This information is stored chronologically and can be incredibly useful for understanding and potentially developing remarkable software.
Chapter 1.4: Creating Type Parameters
Now that we have a solid grasp of interacting with parameters from a user’s perspective, let’s examine how to create these parameters. Typically, parameters are passed to inner constructors. The standard nomenclature for a single type is T. In the following example, x will take on whatever type T is:
mutable struct MyContainer{T}
x::T
function MyContainer(x::Any)
new{typeof(x)}(x)end
end
While this design is effective, it can certainly be optimized for performance. Generally, it’s advisable to restrict field types. For instance, using a subtype operator enhances performance, leading us to write:
mutable struct MyContainer3{T <: Number}
This refinement improves execution speed. In situations where any type is acceptable, I still recommend using T <: Any.
In the case of fields, it's crucial to avoid using abstract types, as seen in the following structure:
mutable struct MyContainer
x::Number
function MyContainer(x::Number)
new(x)end
end
Instead, it would be more effective to utilize a parameterized constructor:
mutable struct MyContainer3{T <: Number}
x::T
function MyContainer3(x::Number)
new{typeof(x)}(x)end
end
Chapter 1.5: Conclusion
While parameters may initially seem complex, their fundamental principles are straightforward and immensely significant. Understanding how to effectively utilize these parameters is crucial, as mismanaging them can lead to substantial obstacles in programming with Julia. Thank you for joining me on this exploration of type parameters!