Creating a Smooth Fade Animation for Text in React
Written on
Chapter 1: Introduction to Fade Animations
In this guide, we will explore how to create a fade-in and fade-out effect for text within a React application. This technique enhances user experience by adding a smooth transition to your UI elements.
This video demonstrates how to achieve fade-in and fade-out animations in React.
Section 1.1: Setting Up the Environment
To begin, we need to incorporate some CSS styles that will facilitate the fade effects. Here’s a simple CSS snippet:
.fade-in {
transition: opacity 1s ease;
}
.fade-out {
opacity: 0;
transition: opacity 1s ease;
}
These class names effectively describe their functions.
Subsection 1.1.1: Creating the Fader Component
Next, we will develop a reusable component named Fader. This component will encapsulate both the UI and the logic necessary for the fade-in and fade-out effects.
- Define Props: The Fader component will accept a text prop, which should be a string. By default, it will display "Hello World!".
Fader.defaultProps = {
text: 'Hello World!'
}
Fader.propTypes = {
text: PropTypes.string,
}
- Import CSS: Ensure that the CSS file is imported into your component (e.g., App.css).
- Initial State: Utilize the useState hook from React to define the initial fade property.
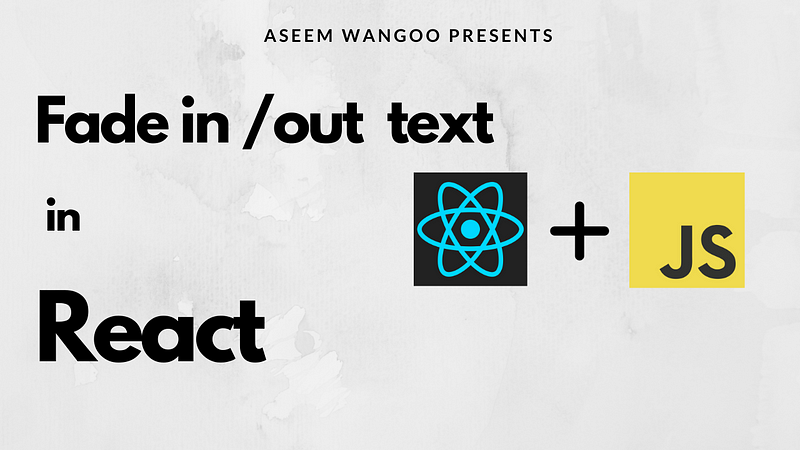
We will set the initial state to fade-in, which will be reflected in the class name of our HTML element.
return (
<>
<h1 className={fadeProp.fade}>{text}</h1></>
)
Section 1.2: Implementing the Fade Logic
To manage the fade-in and fade-out transitions, we will employ the useEffect hook.
useEffect(() => {
const timeout = setInterval(() => {
if (fadeProp.fade === 'fade-in') {
setFadeProp({
fade: 'fade-out'})
} else {
setFadeProp({
fade: 'fade-in'})
}
}, 4000);
return () => clearInterval(timeout);
}, [fadeProp]);
This code snippet utilizes setInterval, alternating the CSS class every 4 seconds based on the current state.
Cleanup Side Effects
It's crucial to clean up side effects in useEffect. If the callback function returns another function, it will be executed before the effect is re-run or when the component unmounts.
return () => clearInterval(timeout);
This line ensures that any existing timer is canceled before a new one begins, preventing potential memory leaks.
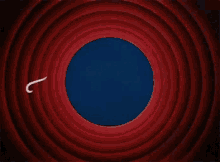
Chapter 2: Conclusion and Additional Resources
This video tutorial provides a comprehensive overview of creating fade animations in React.js.
For more in-depth articles on React, consider exploring the following resources: