Mastering Hapi.js: Static Files and Input Validation Techniques
Written on
Chapter 1: Introduction to Hapi.js
Hapi.js is a lightweight framework for Node.js that facilitates the development of backend web applications. This guide will delve into creating backend services utilizing Hapi.js.
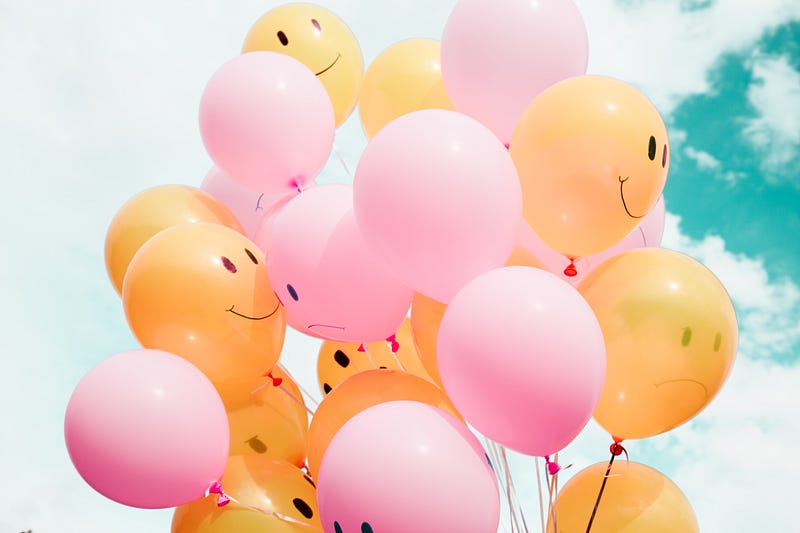
Section 1.1: Configuring Static File Handling
To manage how static files are served, Hapi.js provides various configuration options. Here's how you can set it up:
const Hapi = require('@hapi/hapi');
const Path = require('path');
const init = async () => {
const server = Hapi.server({
port: 3000,
host: '0.0.0.0',
});
await server.register(require('@hapi/inert'));
server.route({
method: 'GET',
path: '/{param*}',
handler: {
directory: {
path: Path.join(__dirname, 'public'),
index: ['pic.png']
}
}
});
await server.start();
console.log('Server running at:', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
In this example, we configure the server to use a specified index file when no other file is provided in the URL.
Section 1.2: Creating a Static File Server
Building a static file server with Hapi.js is straightforward. Below is an example implementation:
const Path = require('path');
const Hapi = require('@hapi/hapi');
const Inert = require('@hapi/inert');
const init = async () => {
const server = new Hapi.Server({
port: 3000,
host: '0.0.0.0',
routes: {
files: {
relativeTo: Path.join(__dirname, 'public')}
}
});
await server.register(Inert);
server.route({
method: 'GET',
path: '/{param*}',
handler: {
directory: {
path: '.',
redirectToSlash: true
}
}
});
await server.start();
console.log('Server running at:', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
In this setup, we define the static file directory using routes.files.relativeTo, allowing us to serve files seamlessly.
Chapter 2: Implementing Input Validation
Input validation is crucial for ensuring that the data received by your server is correct and secure. With Hapi.js, this can be achieved using the @hapi/joi module.
In this video, titled "Hapi Validation and Error Handling," you'll learn effective strategies for handling validation errors in Hapi.js applications.
Here's an example of how to implement input validation:
const Joi = require('@hapi/joi');
const Hapi = require('@hapi/hapi');
const init = async () => {
const server = new Hapi.Server({
port: 3000,
host: '0.0.0.0',
});
server.route({
method: 'GET',
path: '/hello/{name}',
handler (request, h) {
return Hello ${request.params.name}!;},
options: {
validate: {
params: Joi.object({
name: Joi.string().min(3).max(10)})
}
}
});
await server.start();
console.log('Server running at:', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
In this code, we add validation to ensure that the name parameter is a string between 3 and 10 characters. If the input fails validation, the server will respond with a 400 error.
The second video, "Hapi JS Tutorial 1 - Starting Your Server," provides foundational knowledge for setting up your Hapi.js server.
Conclusion
With Hapi.js, you can efficiently validate request parameters using @hapi/joi and serve static file directories using @hapi/inert. These features significantly enhance your backend application's robustness and usability.