Efficiently Sort Numbers with Python: A Comprehensive Guide
Written on
Introduction to Python's Capabilities
Python is an incredibly versatile programming language that spans a wide array of applications, from web development to machine learning. One of its standout features is its capacity to manage extensive datasets, making it a favored choice for data analysis and processing tasks.
Sorting large numbers can often pose significant challenges, but Python provides a robust solution. By leveraging Python scripts for sorting, users can tap into the language's efficiency in handling big data, along with its rich ecosystem of libraries tailored for data manipulation.
Utilizing Python scripts for sorting offers numerous advantages. For starters, it allows for seamless scalability as datasets expand. Moreover, scripts facilitate the automation of data processing tasks, saving time and minimizing the likelihood of human errors.
An added benefit of using Python scripts is the ease with which analyses can be reproduced. This feature enables quick validation of results and necessary adjustments, enhancing the reliability and efficiency of our work. Ultimately, Python's adeptness at managing extensive datasets, combined with its array of tools and libraries, makes it an ideal choice for sorting large sets of numbers in practical applications.
Python Script Overview
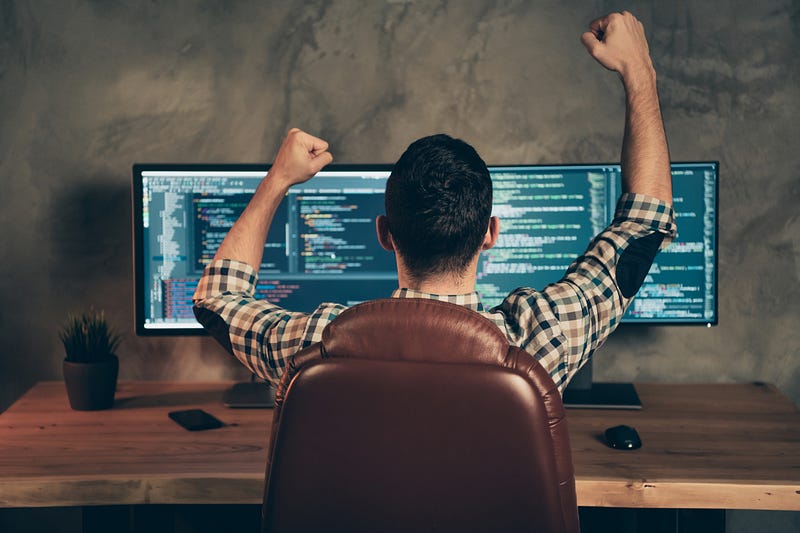
The following script performs two primary functions. It first generates 1,000 random numbers, which are saved in a file named numbers.txt. Subsequently, it accepts command-line arguments, reads these numbers from the input file, sorts them in descending order, and writes the top N numbers to an output file.
import argparse
import random
# Generate random numbers
numbers = [random.randint(1, 1000) for _ in range(1000)]
# Write the numbers to a file
with open("numbers.txt", "w") as f:
for number in numbers:
f.write(f"{number}n")
# Parse the command-line arguments
parser = argparse.ArgumentParser()
parser.add_argument("-n", type=int, required=True, help="Output length N")
parser.add_argument("--input-file", type=str, required=True, help="Path to input file")
parser.add_argument("--output-file", type=str, required=True, help="Path to output file")
args = parser.parse_args()
n = args.n
input_file = args.input_file
output_file = args.output_file
# Read the input file
with open(input_file, "r") as f:
lines = f.readlines()
# Extract the N largest numbers
numbers = sorted([int(line.strip()) for line in lines], reverse=True)[:n]
# Write the result to the output file
with open(output_file, "w") as f:
for number in numbers:
f.write(f"{number}n")
Detailed Steps Explained
- Generation of Random Numbers: A loop iterates 1,000 times, each time creating a random integer between 1 and 1000, adding it to the numbers list.
- Writing to a File: These generated numbers are stored in numbers.txt, which serves as the input for the next phase of the script.
- Command-Line Argument Parsing: The script utilizes the argparse library to manage command-line inputs, including -n (the count of largest numbers to retrieve), --input-file (path to the input file), and --output-file (path for the output file).
- Input File Reading: The script reads integers from the specified input file, compiling them into a list called numbers.
- Sorting: The script sorts the numbers in descending order. For instance, if -n is set to 10, the script will extract the top 10 largest numbers.
- Writing Output: Finally, the sorted numbers are written to the output file defined by the --output-file argument.
Important Considerations
When using this script, keep in mind a few important points:
- The input file must contain one integer per line, while the output file will also list one integer per line in descending order.
- Although the sorted function is efficient for large datasets, consider implementing more advanced sorting algorithms for extremely large data.
- The random library is adequate for basic needs; however, for security-sensitive applications, a more sophisticated random number generator should be employed.
- The script currently lacks error handling; ensure you implement checks for unexpected conditions, such as invalid file formats or missing command-line arguments.
Real-World Applications
This script can significantly simplify the task of sorting large datasets, which is essential across various industries—be it data analysis, processing, or report generation.
For instance, in data analysis, navigating through vast datasets can seem overwhelming. However, organizing the data based on specific criteria makes this task more manageable. By utilizing this script to sort numbers, we streamline the analysis process and store results efficiently.
In data processing, sorting is often the initial step in a workflow. By automating this function, the script allows professionals to redirect their focus toward other critical tasks.
When it comes to report generation, presenting data in an organized manner is crucial. This script assists in sorting data, which can then be incorporated into reports seamlessly.
Professionals across various roles—DevOps Engineers, Data Analysts, Software Developers, and business analysts—can all reap the benefits of this script. By automating sorting tasks, it not only saves time but also reduces the potential for human error. Who wouldn't appreciate a tool that boosts efficiency and minimizes mistakes?
In conclusion, if your role involves sorting large datasets, this script is an indispensable resource. Transition from manual sorting to a more effective and streamlined method of achieving your goals.
Closing Thoughts
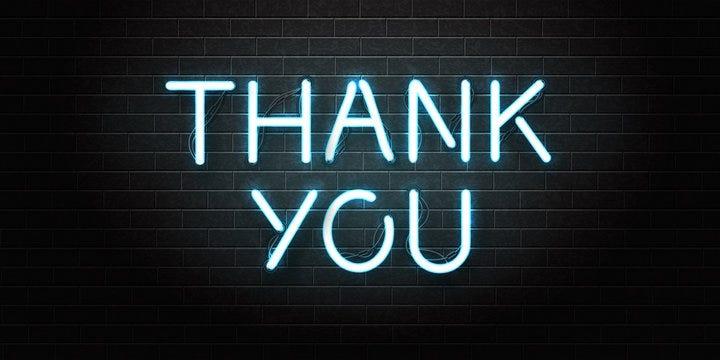
Thank you for engaging with this article! I hope you found the information insightful and valuable. Sorting large datasets is a fundamental task in many fields, and Python simplifies this process significantly. Armed with the scripts outlined here, you can now sort extensive datasets swiftly and effectively.
I believe that learning is a continuous journey, and I appreciate you embarking on this path with me. I encourage you to keep exploring new ideas and concepts and to share your findings with others. Your growth and learning have only just begun!
So, what's next? Continue to challenge yourself, expand your knowledge, and build incredible projects using Python. The opportunities are limitless, and I look forward to seeing what you create next! Thank you for joining me on this adventure, and happy coding!
A demonstration of sorting numbers in a file using Python.
Learn how to read and sort a list of items from a file with Python.